GlobalGateway Image Capture SDK
GlobalGateway Image Capture SDK
Overview:
The Image Capture SDK enables users to capture and upload high-quality ID document images and selfies for identity verification. It further allows for data extraction from the submitted ID document images. It also supports document authentication and facial recognition to verify and authenticate the identity. The SDK leverages a device’s native camera application to acquire images for automatic analysis.
This document contains an overview of the Image Capture SDK, its features, and its minimum system requirements required for implementation. It also provides a detailed description of all functions that developers need to integrate with.
What is Image Capture SDK?
The SDK provides developers with the options needed to integrate their application seamlessly and maintain a customized user experience. The freedom to create any UI with any framework is possible with our pure JavaScript API. This approach allows you to simply call methods in our JavaScript API to capture identity documents (driver’s licenses, ID cards, passports, residence permit), additionally allowing for auto-capture of selfies. This approach also allows complete control over the front end or look and feel of your site. We have also included a sample HTML template to demonstrate how to develop various workflows to get you up and running quickly. Once the image of an ID document is captured, the image is reduced in size, compressed, and passed in RAM to the native application. Images are never stored on the camera roll or any persistent device storage. All image analysis and enhancement takes place in RAM.
NOTE: The SDK may have problems capturing images on desktop or laptop computers due to the lower quality of web cameras.
System Requirements:
The Image Capture SDK is supported on the following platforms:
Browser | Supported version |
---|---|
Safari/iOS | *11.2+ |
Chrome for Android | 67.0+ && Android OS version 4.1+ |
Internet Explorer | Edge 16+ |
Firefox for Android | 63+ |
Chrome for desktop | 67.0+ / Chrome requires HTTPS |
Firefox for desktop | 53+ |
Samsung Internet Browser | 7.2 - 8.2 / 9.2+ |
Other Requirements:
- Devices must have a camera that supports a minimum of 720p resolution.
- Chrome requires the use of HTTPS / SSL when accessing the video for AUTO_CAPTURE mode.
- Browser needs to support getUserMedia
- Browser needs to support Web Assembly
- Browser needs to support WebGL
Unsupported Browsers:
For browsers that do not meet the minimum general requirements listed above, the SDK will return an error and integrators should handle these errors in their application.
Note: The HTML page must run over Https to work / Chrome requires this to access the device's video camera.
Set up for sample app:
Detailed instructions can be found here:
https://developer.trulioo.com/docs/globalgateway-image-capture-sdk-sample-app-installation-guide
The sample app should look like below, you can initialize all different document/selfie capture mechanism with the 2 drop downs.
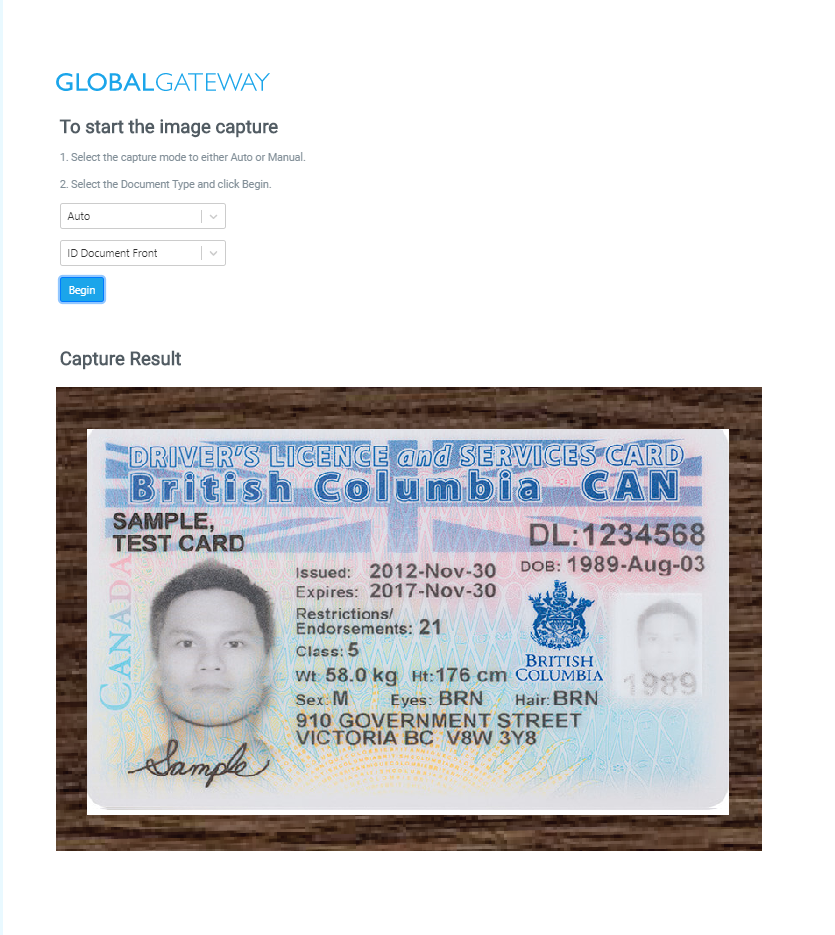
Step 1
GlobalGatewayImageCapture package includes the following items:
- GlobalGatewayCapture.css
- GlobalGateway (folder)
- Images (folder)
- GlobalGatewaySDK (folder, contains helper js files)
- GlobalGatewayImageCaptureSDK (folder)
- GlobalGatewayImageCapture.js
- GlobalGateway-sdk.js
Place Trulioo folder in public folder as it contains helper js files which would be loaded on demand during runtime to reduce footprint.
Include GlobalGatewayCapture.css, ensure the image path are correctly linked to the GlobalGateway/Images folder.
Ensure GlobalGatewayImageCapture.js and GlobalGatewaysdk.js are placed in the same folder. Include only GlobalGatewayImageCapture.js in your html
Step 2
To initialize GlobalGatewayImageCaptureSDK
include
<script type='text/javascript' src="path/to/GlobalGatewayCapturePublic/GlobalGatewayImageCapture.js">
in the BODY of html file.
The SDK will automatically run initialization onload.
Set up Capture Hint Message
Hint messages are set as following:
GLOBALGATEWAY_HEAD_OUTSIDE: 'Place Face in Oval',
GLOBALGATEWAY_HEAD_SKEWED: 'Look Straight Ahead',
GLOBALGATEWAY_AXIS_ANGLE: 'Hold Phone Upright',
GLOBALGATEWAY_HEAD_TOO_CLOSE: 'Move Farther Away',
GLOBALGATEWAY_HEAD_TOO_FAR: 'Get Closer',
GLOBALGATEWAY_STAY_STILL: 'Hold Still',
GLOBALGATEWAY_STOP_SMILING: 'Stop Smiling',
GLOBALGATEWAY_SMILE: 'Smile!',
GLOBALGATEWAY_READY_POSE: 'Hold it There',
GLOBALGATEWAY_NO_FACE_FOUND: 'No Face Detected',
GLOBALGATEWAY_ERROR_GLARE: 'Reduce Glare',
GLOBALGATEWAY_ERROR_FOUR_CORNER: 'Not all document corners visible',
GLOBALGATEWAY_SUCCESS: 'Success',
GLOBALGATEWAY_ERROR_TOO_DARK: 'Too dark. use good lighting',
GLOBALGATEWAY_ERROR_FOCUS: 'Hold Steady',
GLOBALGATEWAY_ERROR_MRZ_MISSING: 'Passport Not Detected',
GLOBALGATEWAY_CV_NO_BARCODE_FOUND: 'No Barcode Detected'
If any of the message need to be changed, set the specific properties of TruliooHints on DOMContentLoaded:
document.addEventListener('DOMContentLoaded', function () {
$('button').attr('disabled', true);
// Instead of "Document Not Found"
GlobalGatewayHints.GLOBALGATEWAY_ERROR_FOUR_CORNER = "DOCUMENT NOT FOUND";
$(':button').attr('disabled', false);
});
**This feature can be used for supporting multiple languages.
Step 3
There are five capture functions along with a stop function in TruliooImageCapture:
- StartDocumentCapture
- StartBarcodeCapture
- StartPassportCapture
- StartGenericDocumentCapture
- StartSelfieCapture
- StopTruliooCapture
After page load, these functions will be loaded to window object.
- StartDocumentCapture will start a capture session to capture any rectangle documents (driver licence, ID cards etc.). It works for both front and back of the document.
- StartBarcodeCapture will start a capture session for barcode capture. Currently this feature only works for the backside of US and Canada driver licence.
- StartSelfieCapture will start a capture session for selfie image. It prompts to smile to ensure the liveness of the user.
- StartPassportCapture will start a capture session to capture the photo page of the passport.
- StartGenericDocumentCapture will start a capture session to capture a generic document like a utility bill
To start capture, call any start function with the following parameters:
(message, timeoutInSecond, isAuto = true, onSuccess = (res) => {}, onError = (err) => {}, retries=0)
- Message (string): message to display during while starting the camera resource
- timeoutInSecond (int): set the length for auto capture. StopTruliooCapture will be called if no image is captured during this period of time. Timeout only works in Auto Capture mode (isAuto=true).
- isAuto (boolean): toggle for Auto Capture mode and Manual Capture mode. When set to manual capture, there will be no timeout triggered.
- onSuccess (function): success handler, which will be called with the captured image in base64 format when an image is successfully captured.
- onError (function): error handler, which will be called with an error object when an error occurs.
- retries (int): optional parameter to set if you would like to track retries in GlobalGateway
Sample usage in index.html.
To manually end the capture section, call StopTruliooCapture().
To verify, set your request body with the byte data after “data:image/jpeg;base64,” prefix:
Using The Captured Image
The result of capture will be in base64 image format:
Image = data:image/jpeg;base64,/9j/4QAYRXhpZgAASUkqAAgAAAAA ....
POST https://api.globaldatacompany.com/verifications/v1/verify
Authorization: Basic ACCESS_TOKEN
Content-Type: application/json
body:
{
"AcceptTruliooTermsAndConditions": true,
"CleansedAddress": false,
"VerboseMode": true,
"ConfigurationName": "Identity Verification",
"CallBackUrl": "www.example.com",
"CountryCode": "US",
"DataFields": {
"PersonInfo": {
"FirstGivenName": "Mary",
"FirstSurName": "Sample"
},
"Document": {
"DocumentFrontImage":"/9j/4QAYRXhpZgAASUkqAAgAAAAA .....IQCEIQCEIQCEIQP/9k=",
"DocumentBackImage": "/9j/4QAYRXhpZgAASUkqAAgAAAAA......LKICIiAiIgIiICIiAiIg//Z",
"LivePhoto": "/9/9j/4QAYRXhpZgAASUkqAAgAAAAA .....JGHGTDKYFRFS/9k=",
"DocumentType": "DrivingLicence"
}
}
}
More information regarding GlobalGateway ID Document Verification step can be found here
https://developer.trulioo.com/docs/document-verification-step-5-verify
Please see below the error messages that are available as part of the SDK.
Error Code | Error message | Description |
---|---|---|
111 | CONSTRAINT_NOT_SATISFIED | The video camera must run at 1280x720, but this device does not support this required resolution. The user is likely using a very old device. You may also see this returned during development because the web app is not being served over TLS. |
112 | NO_CAMERA_FOUND | No video camera was found |
113 | CAMERA_PERMISSION_DENIED | The end user did not click "accept/allow" when prompted for camera permission. Note: If you see this error, call (e.g., ) |
120 | CAMERA_UNKNOWN_DEVICE_IS SU | Unable to start the camera / unknown error |
331 | UNKNOWN_METHOD | An unknown command method was called (e.g., ) |
332 | INVALID_COMMAND_SIGNATURE | Incorrect number of arguments passed to the method |
333 | USER_MEDIA_NOT_SUPPORTED | getUserMedia is not supported by the browser |
334 | WASM_NOT_SUPPORTED | Web Assembly is not supported by the browser |
335 | DEVICE_NOT_SUPPORTED | This device is not compatible with v4.2 |
336 | WEBGL_NOT_SUPPORTED | This device does not support WebGL (specific to Selfie capture) |
339 | WEBGL_SHADE R_NOT_SUPPORTED | This device does not support WebGL shader features (specific to Selfie capture) |
1001 | IMAGE_SMALLER_THAN_MIN | The image is smaller than the minimum size |
1002 | CORRUPT_IMAGE | The image file may not be an image |
1003 | FILE_TYPE_INVALID | The file type is invalid (jpg, png and pdf support only) |
1004 | PDF_FILE_SIZE_OVER_4_MB | The PDF file is over 4MB |
Updated over 3 years ago